tl;dr Is there a simple way (I know, I know!) to map the underlying
I'm running against 1.20.4 so I think I might be falling victim of the issues discussed here:
forums.papermc.io
I feel that this might be a holy grail type question, so please forgive my ignorance if this is either obvious or impossible - or both!
Also I'm not sure of the correct terminology when describing the differences I see between the
What am I trying to do?
I am developing a plugin that creates a bridge between Python and the
For example:
This will be teaching aid - I work in UK Schools and work with (often autistic) kids and my bridge will primarily be used by them. Performance is also not a massive factor for my implementation either.
I'm using reflection and when interrogating the result of a method call I 'sometimes' get
It’s critical for me to be able to map these
Knowing the correct class also allows me to get the methods and fields for that class/interface as well as any parameters and return types. This is essential to allow me to cast from Python to Java data types and back again.
As a simple example, given the interface class:
...which might be returned from a call to get
I need to know that this
Ideally I would like a list of all such maps between
The alternative
I THINK I can create such a mapping myself but it would involve me
I'd then use that in Python to create a manual mapping. But I currently don't know what I don't know - if you see what I mean. What classes are likely to be
I've written a small Python-side script to achieve this for
Note I've normalised the
I'd really like to avoid this approach if I can. Or is this my only sensible option?
Any help, advice or pointers gratefully appreciated!
Thanks for your time.
CraftBukkit
classes to those documented in the Paper
API?I'm running against 1.20.4 so I think I might be falling victim of the issues discussed here:
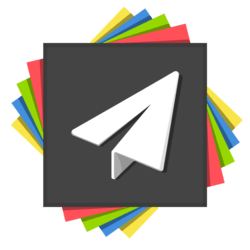
Announcement - Paper & Velocity 1.20.4
The 1.20.4 Update Stable Paper and Velocity 1.20.4 builds have been released! As always, backups are absolutely mandatory. After upgrading your world to 1.20.4, you cannot downgrade back to a lower version! We would like to thank everyone that worked on this update (a lot of people and work...

I feel that this might be a holy grail type question, so please forgive my ignorance if this is either obvious or impossible - or both!
Also I'm not sure of the correct terminology when describing the differences I see between the
Paper
API that I interact with and the CraftBukkit
classes that I get returned. So, I've decided to refer to these as Paper
and CraftBukkit
below. What am I trying to do?
I am developing a plugin that creates a bridge between Python and the
Paper
server. The goal is that most / all of the Paper
server objects will be accessible to Python developers using a Paper
API / Java-like syntax. For example:
Python:
player_location = current_player.getLocation()
block_at_player_location = player_location.getBlock()
log = Material.ACACIA_LOG
block_at_player_location.setType(log)
block_data_at_player_location = block_at_player_location.getBlockData()
This will be teaching aid - I work in UK Schools and work with (often autistic) kids and my bridge will primarily be used by them. Performance is also not a massive factor for my implementation either.
I'm using reflection and when interrogating the result of a method call I 'sometimes' get
CraftBukkit
classes instead of the Paper
ones I'm expecting.It’s critical for me to be able to map these
CraftBukkit
classes to the relevant Paper
class that I can see in the documentation. Being able to identify the returned class and matching it to what I'm expecting in the documentation allows me instantiate the relevant Python class to wrap the returned object. Knowing the correct class also allows me to get the methods and fields for that class/interface as well as any parameters and return types. This is essential to allow me to cast from Python to Java data types and back again.
As a simple example, given the interface class:
org.bukkit.craftbukkit.v1_20_R3.block.impl.CraftRotatable
...which might be returned from a call to get
BlockData
on a Block
of type Material.ACACIA_LOG
.I need to know that this
CraftBukkit
interface class is “equivalent” to the BlockData
interface in the Paper
world named:org.bukkit.block.data.Orientable
Ideally I would like a list of all such maps between
CraftBukkit
classes / interfaces / objects / Enums and Paper
objects. ASSUMING, that is, that they have a one-to-one, identical purpose relationship.The alternative
I THINK I can create such a mapping myself but it would involve me
- writing code to create "stuff" in the Minecraft world using a known
Paper
class / interface - interrogating the classes that actually get returned
- outputting the mapping to a log file
- copying the output into code structures
I'd then use that in Python to create a manual mapping. But I currently don't know what I don't know - if you see what I mean. What classes are likely to be
CraftBukkit
and what are currently Paper
?I've written a small Python-side script to achieve this for
BlockData
. Here's a sample of the map between Paper
and CrafftBukkit
BlockData
interface classes. Note I've normalised the
CraftBukkit
interface class by removing the version number.
Python:
block_data_class_map = {
'org.bukkit.block.data.type.Switch': 'org.bukkit.craftbukkit.block.impl.CraftButtonAbstract',
'org.bukkit.block.data.type.Door': 'org.bukkit.craftbukkit.block.impl.CraftDoor',
'org.bukkit.block.data.type.Fence': 'org.bukkit.craftbukkit.block.impl.CraftFence',
'org.bukkit.block.data.type.Gate': 'org.bukkit.craftbukkit.block.impl.CraftFenceGate',
'org.bukkit.block.data.type.HangingSign': 'org.bukkit.craftbukkit.block.impl.CraftCeilingHangingSign',
'org.bukkit.block.data.type.Leaves': 'org.bukkit.craftbukkit.block.impl.CraftLeaves',
'org.bukkit.block.data.Orientable': 'org.bukkit.craftbukkit.block.impl.CraftRotatable',
...
...
...
'org.bukkit.block.data.type.TurtleEgg': 'org.bukkit.craftbukkit.block.impl.CraftTurtleEgg',
}
I'd really like to avoid this approach if I can. Or is this my only sensible option?
Any help, advice or pointers gratefully appreciated!
Thanks for your time.
- Version Output
-
This server is running Paper version git-Paper-389 (MC: 1.20.4) (Implementing API version 1.20.4-R0.1-SNAPSHOT) (Git: 848a396)
You are 20 version(s) behind
Download the new version at: https://papermc.io/downloads/paper