Hello, I am trying the following:
API version 1.21.1-R0.1-SNAPSHOT (tried originally with 1.20.4)
The results in-game:
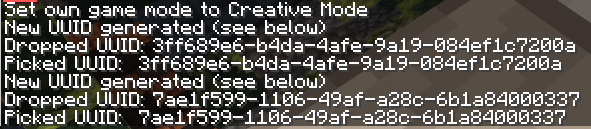
Interestingly, the item keeps the UUID when it's on the ground and it's picked up again. It simply gets reset to null after I drop it again.
It looks like it loses the PDC after I throw it twice, or in the transition between being in the inventory and then dropped.
Is this due to listening to the wrong events? Or perhaps items in inventory are different from items on the ground and they don't share data between them?
In the following guide:
docs.papermc.io
there is a section that warns the following:
Thanks for any help
API version 1.21.1-R0.1-SNAPSHOT (tried originally with 1.20.4)
Java:
@EventHandler
public void onItemPickup(EntityPickupItemEvent e) {
PersistentDataContainer container = e.getItem().getPersistentDataContainer();
UUID id = container.get(ns_key, uuid_t);
if(id == null) {
id = UUID.randomUUID();
container.set(ns_key, new UUIDDataType(), id);
}
}
@EventHandler
public void onItemDrop(PlayerDropItemEvent e) {
PersistentDataContainer container = e.getItemDrop().getPersistentDataContainer();
UUID id = container.get(ns_key, uuid_t);
if(id == null) {
id = UUID.randomUUID();
container.set(ns_key, uuid_t, id);
}
}
The results in-game:
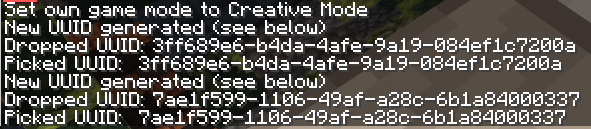
Interestingly, the item keeps the UUID when it's on the ground and it's picked up again. It simply gets reset to null after I drop it again.
It looks like it loses the PDC after I throw it twice, or in the transition between being in the inventory and then dropped.
Is this due to listening to the wrong events? Or perhaps items in inventory are different from items on the ground and they don't share data between them?
In the following guide:
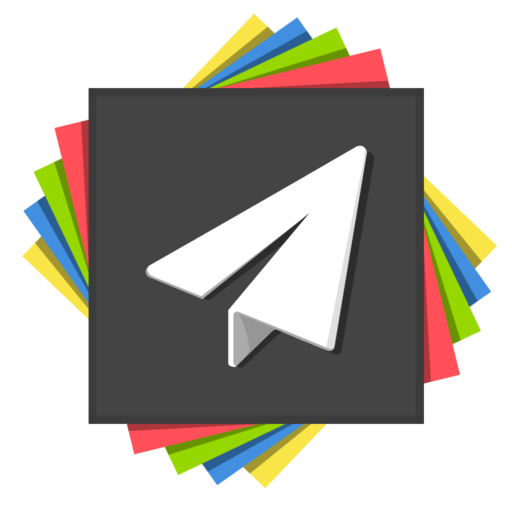
Persistent Data Container (PDC) | PaperMC Docs
A guide to the PDC API for storing data.
But this raises more questions for me: I assume items on the ground AND on the inventory are the same type, right? In the case they weren't, how am I supposed to copy PDC data from one to the other? Even so, since the PDC data is kept from the dropped to picked up state, I guess this is not the case...CAUTION
Data is not copied across holders for you, and needs to be manually copied if 'moving' between PersistentDataHolders.
E.g. Placing an ItemStack as a Block (with a TileState) does not copy over PDC data.
Thanks for any help
- Version Output
- This server is running Paper version 1.21.1-132-ver/1.21.1@b48403b (2024-11-21T10:14:27Z) (Implementing API version 1.21.1-R0.1-SNAPSHOT)